1. نصب SDK RedStone
ابتدا باید SDK مرتبط را در پروژه خود نصب کنید. از دستور زیر استفاده کنید:
npm install @redstone-finance/evm-connector
2. نوشتن قرارداد هوشمند
یک قرارداد ساده برای استفاده از دادههای قیمت اوراکل بنویسیم:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@redstone-finance/evm-connector/contracts/DataReceiver.sol";
contract PriceConsumer is DataReceiver {
function getPrice() public view returns (uint256) {
bytes32 dataFeedId = bytes32("ETH");
uint256 ethPrice = getDataFeedValue(dataFeedId);
return ethPrice;
}
}
3. تنظیم محیط برای دیپلوی
در پروژه خود یک فایل hardhat.config.js ایجاد کنید و شبکه آزمایشی مثل Rinkeby یا Sepolia را اضافه کنید:
require("@nomiclabs/hardhat-waffle");
module.exports = {
networks: {
rinkeby: {
url: "https://rinkeby.infura.io/v3/YOUR_INFURA_PROJECT_ID",
accounts: ["YOUR_PRIVATE_KEY"]
}
},
solidity: "0.8.0"
};
4. دیپلوی قرارداد
یک فایل اسکریپت برای دیپلوی ایجاد کنید، بهعنوان مثال scripts/deploy.js:
async function main() {
const PriceConsumer = await ethers.getContractFactory("PriceConsumer");
const priceConsumer = await PriceConsumer.deploy();
console.log("Contract deployed to:", priceConsumer.address);
}
main()
.then(() => process.exit(0))
.catch((error) => {
console.error(error);
process.exit(1);
});
سپس با استفاده از Hardhat قرارداد را دیپلوی کنید:
npx hardhat run scripts/deploy.js - network rinkeby
5. تست قرارداد
پس از دیپلوی، از طریق یک فایل تست یا اسکریپت دیگر میتوانید عملکرد قرارداد را بررسی کنید و دادههای قیمت را فراخوانی کنید.
async function main() {
const priceConsumer = await ethers.getContractAt("PriceConsumer", "YOUR_CONTRACT_ADDRESS");
const ethPrice = await priceConsumer.getPrice();
console.log("ETH Price:", ethPrice.toString());
}
main();
این مراحل به شما کمک میکنند تا SDK RedStone را نصب کنید، قرارداد هوشمند خود را دیپلوی کنید و آن را روی شبکه آزمایشی مانند Sepolia تست کنید.
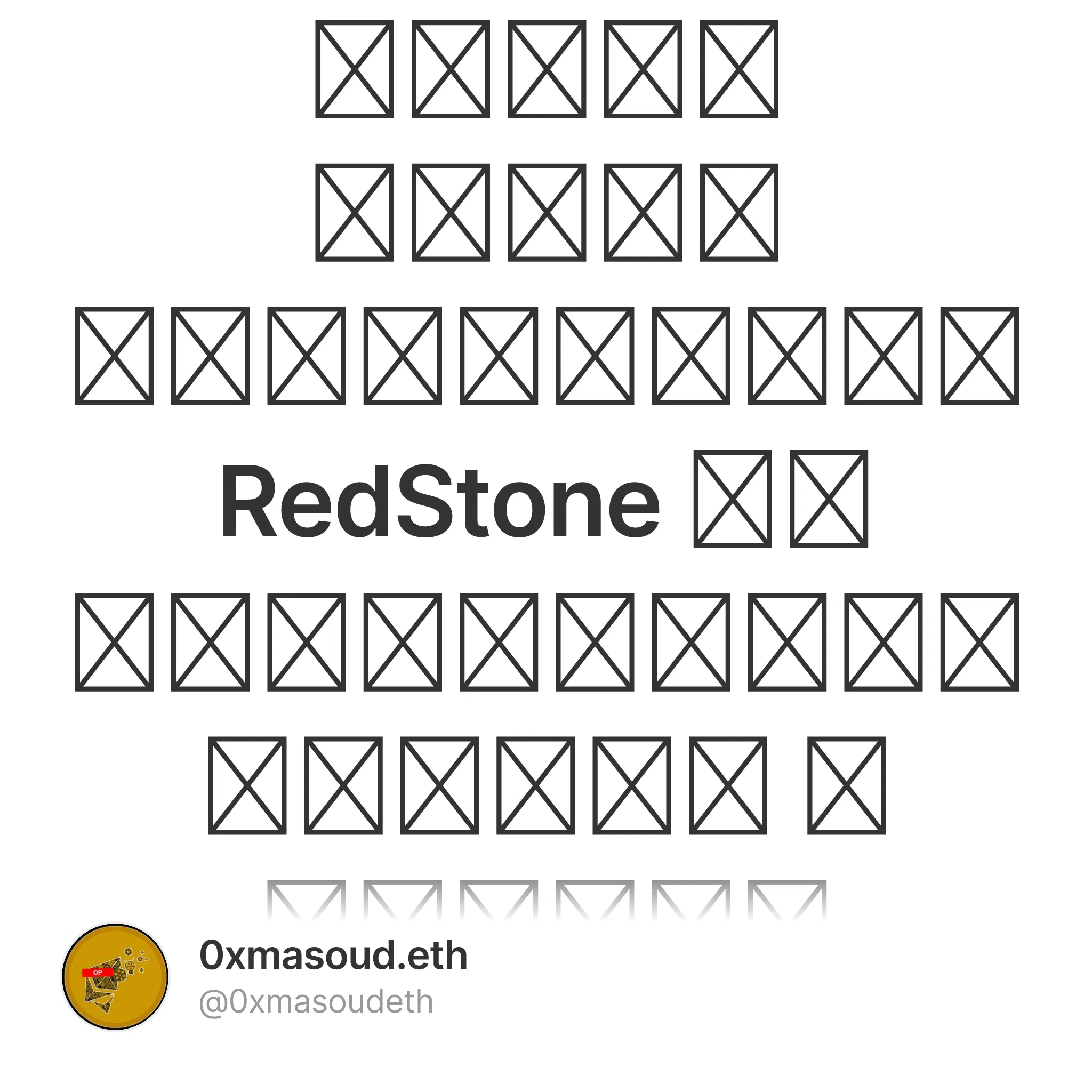
Collect this post to permanently own it.

Subscribe to 0xmasoud.eth and never miss a post.